If you’re new to programming and new to the concept of what a software program is, let’s start there. According to Techopedia, “A software program is commonly defined as a set of instructions, or a set of modules or procedures, that allow for a certain type of computer operation.” A program’s instructions can be written in several languages including, of course, Java. But how exactly does Java work?
How Java Works (in a nutshell)
Java works by first compiling the source code into bytecode. Then, the bytecode can be compiled into machine code with the Java Virtual Machine (JVM). Java’s bytecode can run on any device with the JVM which is why Java is known as a “write once, run anywhere” language.
Of course, this is a slightly simplified version of how Java works. There’s actually much more to it. On a technical level, when writing a simple “Hello World” program with Java, the data makes several stops before it ends up as text on a screen. A more thorough explanation is given, almost comically, by my fellow University student; Sohail Ahmed Ansari.
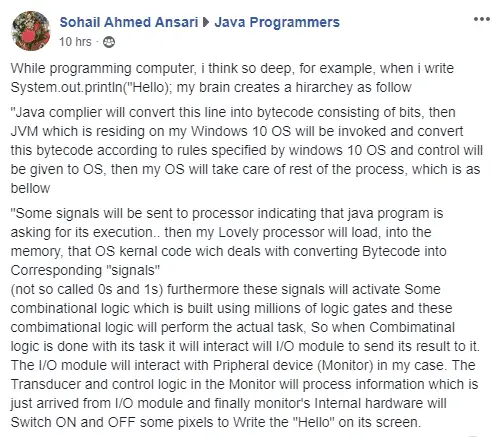
Sohail actually goes one step further and explains not just how Java programs work, but how software programs work as a whole as they interact with the Operating System (OS). The latter part of his explanation is true of all programs. However, in order to truly understand how Java works and why it’s unique, let’s first take a look at how another language works.
How C++ works
When Java was created in 1995, it was modeled after C++. There are some similarities in these languages and some differences. Of course, the syntax is different. However, the main difference lies in how the code is executed. Unlike Java, which is compiled into bytecode, which can then run on any device that has the JVM, C++ is compiled directly into machine code. This is why Java is famously known to be “Write once, run anywhere.”
When a program written in C++ is compiled, that compiled code can only run that environment. In order for the same program to run on another environment, the source code must be transferred to that environment and then compiled. This is one of the advantages that Java has over other languages. Let’s take a look at Java’s JVM and see what makes it so special.
The JVM and Machine Code
We’ve already established that C++ only runs on the environment in which it was compiled. The Java Virtual Machine provides the runtime environment needed for Java to work on virtually any computer. Once the Java program is compiled into bytecode, it can then be interpreted into machine code through the JVM.
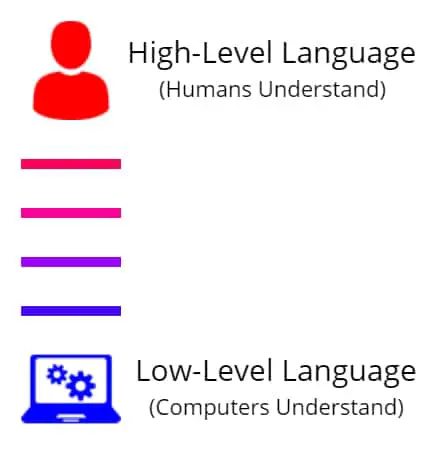
This works in such a way because most computers have their own machine code. Machine code, if you’re not already familiar, is code that only the computer can understand. It’s different from high to mid-level code that we use to actually write a program. Machine code is also referred to as assembly or low-level code. The lower the level, the closer the code is to actual instructions for the processor. The higher it is, the closer it is to something humans can understand.
Object-Oriented Programming
Up until now, I’ve described how Java works behind the scenes. However, it’s also important to understand how Java works on the front end. Java is known to be a general-purpose, Object-Oriented Programming (OOP) language. General-purpose simply refers to Java’s vast capability. There’s isn’t much you can’t do with Java. Object-oriented on the other hand refers to the fact that virtually everything in Java is an object.
I won’t dive too deeply into OOP here. However, I’ll briefly describe in basic terms what an object is. An object in OOP is like an object in real life. This can refer to a person, place, or thing. Just as I have two arms and two legs which you might refer to characteristics, if I were an object in a program, these would be referred to as data types. Also, just as I’m capable of doing things in real life, the object that represents me in the program can also do things. These actions are types of operations, also known as functions. Objects have states (attributes or characteristics), behaviors (functions), and identities (the name of the object).
Java Classes, Methods, and Variables
When writing a new program in Java, you must declare a class name. As a beginner, this is all you need to know. However, after you master the fundamentals of variables and methods, you’ll have to take a deeper look at OOP, classes, and some of the more advanced topics. For now, I’ll tell you that classes are essentially objects. Or rather, they’re the blueprint for the objects which houses everything that define it. The variables refer to the state of the object and the methods refer to the behavior.
As you’re starting out learning Java, you will likely only use one object: the main class. However, after you learn the fundamentals and reach OOP, you will have multiple classes interacting with each other. Remember, the method of an object refers to its behavior. Therefore, when objects interact with each other, they’re simply calling said object’s method for such behavior.
Related Articles