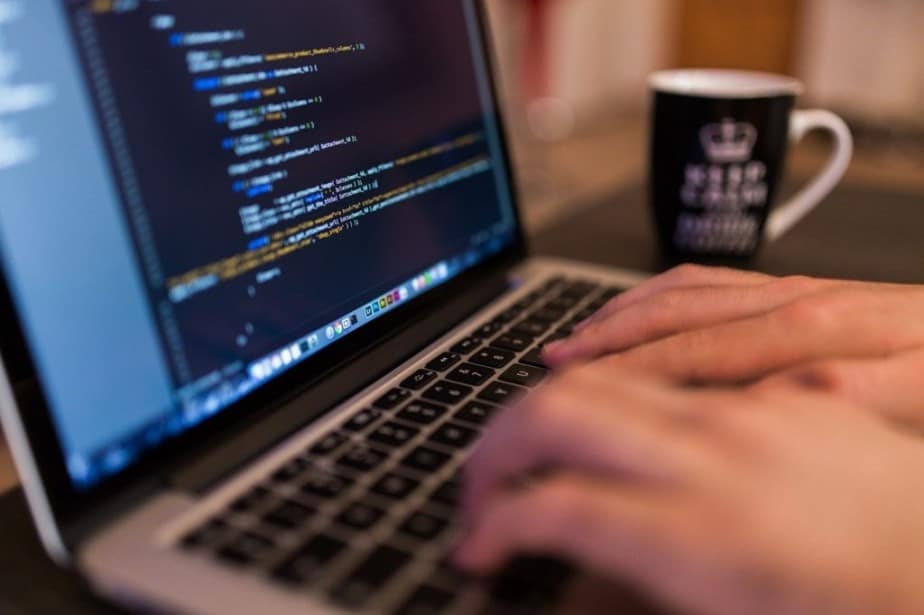
If you’ve decided to major in Computer Science (C.S.) then you’ve come to the right place. I’ve outlined all the Computer Science subjects that are in a core C.S. course load.
Also, I want to congratulate you because you’ve just made an excellent career choice. Computer Science and its various fields are constantly growing, yet there are not enough talented people to fill the jobs. However, there is a growing number of students majoring in C.S. apposed to other STEM fields. Rightfully so if you ask me. Now let’s jump into the core Computer Science Subjects.
Computer Science Subjects:
Intro Computer Science
Computer Networks
Calculus
Data Structures and Algorithms
Database Systems (DBMS)
Discrete Structures
Java
Python
Object-Oriented Programming
Operating Systems
Mobile Development
Web Development
Computer Science
The first subject on the list is a general overview of Computer Science and briefly covers everything you can expect to learn up until you graduate. It provides an overview of the subjects, teaches basic problem-solving methods, and answers many of the questions you’ll have, including “what is Computer Science?”
Computer Science (C.S.) is the theory of computation as well as the broad study of computers. Computer Science, at its core, is the computation and manipulation of data to solve real-world problems. As a field of study, C.S. subjects consist of hardware, software engineering, networks, database management systems, operating systems, algorithms, and many others. These subjects also fall under different categorizations.
Theoretical Computer Science (TCS) focuses more on mathematical computation, programming theory, data structures, algorithm analysis, and many other topics. TCS is an incredible part of Computer Science because it focuses on computation for its own sake, regardless of particular implementations.
Computer Systems encompasses computer networks, database management (DBMS), computer architecture, security and cryptography, and more. Software engineering involves the design, implementation, and modification of software at the highest level. Software engineering in itself has various paradigms, including Object-Oriented Programming (OOP).
Computer Networks
Computer Networking is another fundamental Computer Science subject and it was unexpectedly one of my favorite subjects. Networking included an overview of network architectures, layered architectures, routing and congestion control, protocols, Open System Interconnection (OSI) reference model, local area networks (LAN), and network security.
In the networking class offered at the university I attend, we studied these topics using the largest computer network in the world as our example: the internet.
We use it every day and yet we take for it for granted. In studying the internet, we can learn about the vast protocols set in place, the various network layers, how each node interacts, and much more. In the video below, Vint Cerf, the co-creator of the internet explains what the internet actually is.
Calculus
Calculus is a required Computer Science subject at any University at some level. At Governors State University (GSU), only Calculus 1 is required. However, at Purdue Northwest University (PNW), where I used to attend, Calculus 1, Calculus 2, and Calculus 3 were required. Depending on which field you intend to enter once you complete your degree, the addition Calculus could be very beneficial.
I personally found Calculus to be difficult, but that was only because I didn’t have the best foundation of Algebra and Trigonometry, which is crucial to success in Calculus. Although challenging, Calculus can be very fun and rewarding to learn. Calculus 1 is also known as Differential Calculus because the primary subject matter involves finding rates of change using derivatives.
As you progress to Calculus 2, you learn about anti-derivatives, also known as integrals. Integral Calculus deals with the area under a curve which is essentially cumulative change. Calculus 3, also known as Multivariable Calculus, applies the concepts previously learned to 3-dimensional models.
Data Structures & Algorithms
Data Structures and Algorithms are sometimes two different subjects, but they go hand in hand with each other. This is an intermediate programming subject that introduces more advanced problem solving concepts and techniques. Data Structures and Algorithms emphasizes real-world programming issues such as reliability, efficiency, and complexity.
Algorithms are simply a set of rules to be followed in order to solve a particular problem. The problem at hand is often some form of data manipulation. This is where data structures come in handy. Data structures then become tools to assist us in the creation of complex algorithms.
This class was challenging but it really took my programming skills to another level. It’s also important to note that this is one of the most important Computer Science subjects to learn if you want to get a job as a software developer. Especially if you want to work at a large tech company such as Facebook, Amazon, Netflix, or Google (FANG). Their interview processes are weighed heavily on these concepts.
Database Systems (DBMS)
Database Systems, also referred to as Database Management Systems (DBMS), focuses on the practical development of databases. This subject further enhances a programmer’s ability to design and implement databases. To be more specific, Database Systems covers concepts of data independence, data redundancies, data modeling, relational modeling, building relationships, different query languages such as SQL and QBE, and more.
If you’re not familiar with what a database is, it’s a storage of a large variety of structured data such as usernames or passwords. Database Management Systems are the software that accesses and controls the databases. There are several types of database models, each with its benefits and functionality. Relational databases seem to be the most common in the real world, as far as I’ve seen.
Discrete Structures
Discrete Structures is another math subject but unlike Calculus, Discrete math has a greater focus on Computer Science related mathematics. For example, Discrete Structures covers the logic, probability, recursion, graphs, trees, and other math-related theoretical C.S. topics. Where Calculus describes models that change continuously, Discrete math describes models that have sequential steps.
Another thing that separates Discrete Structures from Calculus is that it’s a little more fun. I know, that’s very opinion-based. However, I’m not the only one who holds this opinion. Because it’s based more on problem-solving and logic than Calculus, it just feels like more of a game to complete assignments. That said, it’s still a challenging course and should not be taken lightly.
If you’re interested in learning more about Discrete Math, Saylor Academy has a complete university-level course free of cost. It also might not be a bad idea to check it out if you’re taking this next semester.
Java
Java, although decreasing in popularity, is still the most popular programming language in the world. Most universities teach one of three different languages to introduce fundamental programming concepts: Java, Python, or some variation of C. In my case, Java was the first. When I was first getting started with it, I’m not going to lie, I found it pretty challenging. Eventually, though, I got the hang of and I started to love it.
If you take Java, the course you take may be called ‘Intro to Java’ but Java in itself isn’t really the focus of this Computer Science subject. Java is the tool used in the class but the real focus is on programming fundamentals leading up to OOP (Object-Oriented Programming). The reason for this is that most languages are fundamentally the same but syntactically different. Thus, if you learn the fundamentals of one language you can easily learn the next.
Python
Python is another popular general-purpose programming language similar to Java. As a subject, it covers the same fundamentals as Java up to and including Object-Oriented Programming, as well as basic data structures and algorithms. As a language, Python is thought to be easier to learn than Java because it has a shorter syntax.
Certain features of Python make it a great choice for web development, machine learning, data processing, scientific computing, and more. As it increases in popularity, Python is taught in more and more Universities. In fact, in recent days Python has become even more popular than Java in education.
Object-Oriented Programming
Object-Oriented Programming is an introductory Computer Science subject centering around the OOP paradigm. Programming paradigms simply refer to a style or methodology of programming. In object-oriented programming, the fundamental building blocks of the program are thought of as objects, similar to objects in the real world. These objects can contain various data sets.
For instance, a real-life object such as a person can have a specific height, weight, age, status, etc. Similarly, a player in a video game can have hit points, a player level, and other stats. Certain programming languages, such as Java, Python, and C++ are OOP languages and others, such as C, Basic, and a few others are not.
Another important thing to note about OOP is that it’s only one of many programming paradigms. There are other paradigms such as procedural, logical, and functional programming. No one paradigm is the best overall just as no one programming language is the best. However, given an individual problem, there is likely a ‘best paradigm’ and a ‘best language’ to solve said problem, depending on various factors.
Operating Systems
Operating Systems (OS) are a core part of computers as well as an essential subject to learn. An OS is the main software in a computer that manages all other software and as well as the hardware. You’re probably aware of the most popular operating systems such as Windows, Android, macOS, Linux, Chrome OS, and a few others.
As a subject, operating systems covers the functionality and architecture of operating systems such as OS design, computer system structure, process management, memory management, I/O management, security, and more. I haven’t taken this class yet but I’m told it can be challenging, especially when you dig into the more advanced courses.
Mobile App Development
At my university, Mobile Development is Android-based, as opposed to IOS-based. This means that Java was the language at the core of the program instead of C. We use Eclipse IDE alongside Android SDK as well as additional frameworks to create mobile apps.
This is another subject that I personally haven’t taken yet, so I can’t speak to its level of difficulty or what was particularly enjoyable. However, I can say that I’m looking forward to learning mobile application development and eventually creating my own mobile apps.
Flappy Bird always comes to mind when I think about mobile apps. If you’d like to create a mock version, there’s a full video tutorial for that and 9 other beginner-level projects here. It’s not a mobile application, but it’s great practice if you’re learning Java and getting familiar with the syntax.
Web Development
Web development is perhaps my favorite Computer Science subject. When developing a website, I feel like less of a computer scientist and more of a computer artist. Web dev is a starting point for many who want to learn how to “code” because learning how to build a basic web page with HTML and CSS is easier than learning the basics of functional programming and OOP with Java. I can personally attest to this, given that my interests also began with web development.
Courses in web development always begin by teaching how to build a website “skeleton” with HTML and then styling it with CSS. Once these foundational concepts are learned, more complex languages such JavaScript can be added to give a website more functionality.