I’ve been programming with Java for more than a year now but I’ve never really stopped and asked myself, “What is Java?”
I know that it’s a programming language and all, but I realized I didn’t know much more than that.
So I put together this complete guide to answer that question and subsequent questions in full. Now let’s learn what Java really is!
What Is Java?
Java is a general-purpose, object-oriented programming language similar to Python and C++. However, Java has some distinct differences. For example, Java is platform-independent which means it can run on any device that has the Java Runtime Environment.
Components of Java
There are several components to the Java programming language that allow developers to create applications and allow clients to run applications.
These components include the JVM (Java Virtual Machine), JRE (Java Runtime Environment), and the JDK (Java Development Kit).
- JVM (Java Virtual Machine): The virtual machine that provides the runtime environment.
- JRE (Java Runtime Environment): Java is first compiled in bytecode. The JRE reads in the bytecode from the client-side and further compiles it to machine code.
- JDK (Java Development Kit): The development suite necessary to develop Java applications and includes the JVM and JRE in download.
What Is Java Used For?
Java is used to create web and mobile applications as well as smaller applications that run on web pages referred to as applets. Java is used in smaller applications as well as large-scale projects the likes of which you’re certainly familiar with.
For example, Java was used in the creation of Google’s search engines, Amazon’s online store, YouTube’s video platform, and much more. Java can also be used to create small banking applications or games that are perfect for beginners.
Check out these 10 Java projects for beginners with full tutorials. This way, you can learn how to create these applications from scratch. This is the best way to learn Java in my opinion.
Is Java Hard To Learn?
I actually did a study on this question and the results might surprise you. I asked 324 Java programmers and roughly 75% said that it was NOT a hard language to learn.
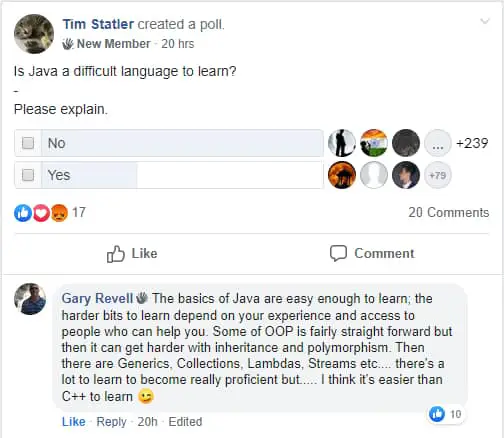
Since Java is often compared to Python and C++, there’s another way to determine the difficulty of Java. This is by determining how easy or hard it is relative to these two languages.
It’s commonly held that Java is more difficult to learn than Python but easier to learn than C++.
How Is Java Different From Other Languages?
I’ll start with the big one: Java and JavaScript are two totally different languages, they’re very different from each other.
That said, the main difference between Java and other languages such as C++ is that Java is platform-independent. This means that Java code can pretty much run the same on any device.
The main difference between Java and Python is that Python is a bit easier to code with, yet Java is a bit quicker than Python.
Language | Object-Oriented | Platform-Independent | Speed |
---|---|---|---|
Python | ✔️ | ✔️ | Slow |
Java | ✔️ | ✔️ | Medium |
C++ | ✔️ | ❌ | Fast |
Features Of Java
There are 12 features that describe the Java programming language. These features are important to know as a beginner.
For many Computer Science students, Java is the first language they learn. Understanding these features will help you to succeed in your course, and help you will future courses.
Unlike other languages such as the C languages, Java is platform-independent. This is probably the most notable feature of Java. But what exactly does that mean?
To put it simply, this means that Java can run on any device. It’s able to do so because of the Java Virtual Machine (JVM). This is what gives credence to the common motto for Java which is “Write once, run anywhere.”
With the C languages, the program is compiled and it can then run on that Operating System (OS).
Java, on the other hand, is compiled into bytecode which can run on any machine with the Java Runtime Environment (JRE). This includes Linux OS, Windows OS, and Mac OS.
2. Portable
Java is also Portable, which is very closely related to the platform-independent feature of Java.
At first, I was having trouble distinguishing between the two. Platform-independent means a program can run across different platforms, i.e. Operating Systems and hardware.
Portability, on the other hand, means a program can run not only across different platforms but also across different environments, which extend beyond the hardware and OS.
3. Object-Oriented
Object-Oriented Programming (OOP) is another defining feature of Java. It refers to a language, such as Java, that uses objects.
The term ‘object’ refers to any variable in the language that is given a name (identity), data (attribute), and a behavior. If you’re familiar with Java at all, you would know that we use objects all the time.
They’re the core building blocks of Java. There are six OOP concepts; Abstraction, Class, Encapsulation, Inheritance, Object, and Polymorphism. You can learn more about OOP here:
Oracle: Object-Oriented Programming
4. Simple
Another feature of Java is its simplicity. It’s not quite as easy to learn as the Python programming language, but it’s much easier than the C++ programming language.
Also, Java has a very simple and easy to use syntax since it’s based on C++. It’s done away with unnecessary features such as pointers and operator overloading.
Yet, despite eliminating these features, Java still has more functionality due to its large class library.
5. Secure
The security features of Java happen to correspond with the simplicity features.
What I mean is that as a result of Java eliminating features such as pointers, Java has become a more secure language. But it’s not just the absence of pointers that makes Java safe.
Additionally, the Java Virtual Machine (JVM) adds a level of security in and of itself. Java also has a security manager that verifies which resources a class can access.
6. Robust
Another buzzword used to describe Java is robust. Java is robust in the sense that it is both powerful and reliable.
It has several features that allow us to consider it robust. One such feature is garbage collection, which finds unused objects that are no longer needed and deletes them to free up memory.
Another is that it checks for bugs early on, contributing to far fewer errors, which speaks to Java’s reliability.
7. Dynamic
Java is strictly static in terms of its compiler. Yet, Java’s runtime system and the language itself are dynamic in their linking stages.
This means that classes aren’t unnecessarily linked, rather, they’re loaded on demand.
The automatic memory management feature, known as garbage collection, also attributes to Java’s Dynamic nature.
8. Distributed
Another feature of Java is that it’s distributed. Distributed programming allows us to distribute our application across two or more computers.
Java does this using technology such as the Remote Method Indication (RMI) mechanism, the Common Object Request Broker Architecture (CORBA), and Enterprise JavaBeans (EJBs).
You can learn more about these technologies and how they’re used here:
Oracle: Distributed Java Programming with RMI and CORBA
9. Multi-Threading
Multi-threading is a feature in Java that allows for multiple parts of a program to be executed concurrently, thus optimizing the CPU.
Each thread then is like a separate program. Since these threads run concurrently, they share a common memory area.
Threads can be created by either extending the thread class or by implementing the runnable interface.
Multi-threading is used in animation, games, and other multimedia. You can learn more about multi-threading here:
Java T Point: Multi-Threading in Java
10. Architecture Neutral
When Java is described as Architecture Neutral, that means that not only will it run on any environment, but it will run exactly the same.
This is because unlike other languages such as C++, primitive variables in the Java language such as int are fixed.
The int value won’t vary in size when running in a different environment.
11. High Performance
With the Just-In-Time (JIT) feature, Java is without question high-performance.
This feature of Java stems yet again from the JVM converting bytecode to machine code.
The JIT compiler works with the JVM and optimizes the conversion of bytecode to native machine code.
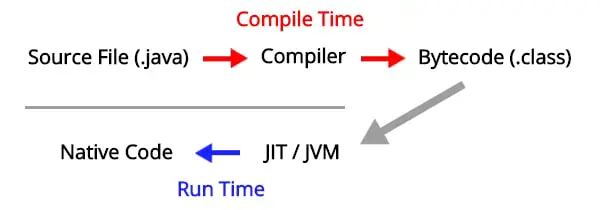
12. Interpreted
Java is an interpreted programming language for the simple fact that it’s not subject to being compiled into machine code before it’s distributed.
Once Java is converted to bytecode, it can be compiled ahead of time, or it can be interpreted on the fly using the Java Virtual Machine (JVM) and the Just-In-Time (JIT) compiler.
Therefore, Java is both a compiled language and an interpreted language.